Someplace New
A few weeks ago I got a shiny new Adafruit FLORA kit in the mail, it’s a lovely little board, and a lot of thought and care has gone into its design. Here’s what came in the box:
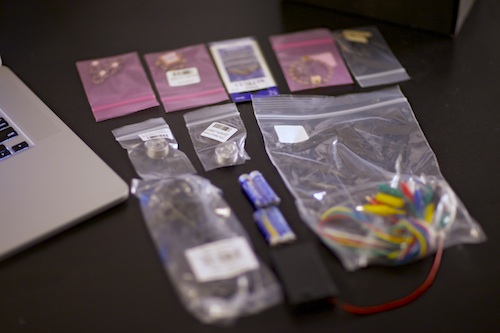
That’s one GPS input, and bunch of LED outputs, prototyping cable, and a controller board. I was most excited about the LED modules. They solve a bunch of the problems associated with attaching LEDs to garments and getting them to produce some useful color in a controllable fashion.
I’ve been toying around with wearables for a while, and it has always suffered from a utility & UX problem. Other than glowing for the sake of glowing (which can be quite beautiful), there’s a short number of projects that meet nicely at the intersection of aesthetics & function. Combining a shirt with a computer has a tendency to produce a slightly uncomfortable shirt and an unreliable computer.
It’s not to say there isn’t really cool and exciting work being done, and some of it can be found documented on Syuzi Pakhchyan’s Fashioning Technology blog. When I think about wearables technology, it roughly breaks down for me into:
- Blinking Lights.
- Location tracking.
- Ambient sensing.
- Materials research.
- Fabrication methods.
Most of the things I find to be really interesting tend to lean more into the ambient sensors category, largely because input technologies tend to lag output technologies in resolution/bandwidth/cost, and so they get less play time. One old project I put together was a top hat with a magnetic hal sensor, it would change color depending on which way one was pointed. It was pretty useless, most of what I learned from the experiment was that people really don’t like staring at super-bright color changing LED when trying to talk to you. Eventually I covered the LED with a translucent toy rubber frog, the magic hat was much improved.
I digress.
As the FLORA was a gift from Adafruit, I wanted to see if I could find something to do with it that would show the system off. Having recently lost my sewing lab, and living in a tiny apartment, it also turned into an experiment into what I could make with minimal resources. No space to pattern out a jacket from scratch.
The first idea I had was to put a couple of color changers under the collar of a dress shirt. San Francisco has recently seen the arrival of urban clothing retailer UNIQLO, which is like a Japanese version of the GAP. It’s the perfect art supply store for clothing moders and hackers. We braved the pre-holiday madness, but where unable to find a suitable dress shirt. At least not one I would wear in public. Dress shirts also suffer from the problem of needing regular cleaning, a part of the fashion tech lifecycle that’s a little rough around the edges. Instead I picked up a nice black cotton blazer on sale for $39, a price at which I could afford to make mistakes without crying too hard. In addition, cotton is easy to hand sew, and very forgiving if one needs to back-out stitches.
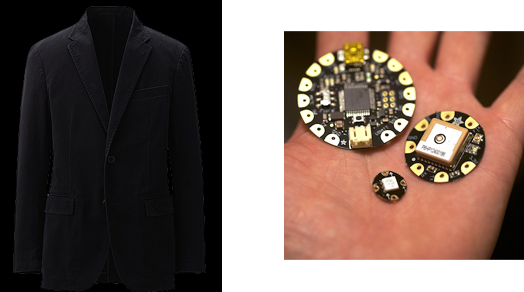
First step was testing the setup. Using the included aligator clip jump clips, it just takes a few minutes to get the board wired up. I downloaded the Adafruit Arduino app, updated the GPS libraries and LED libraries from the Adafruit github repo, and ran the test examples. GPS lock and blinkin’ lights achieved!
Sewing was a little harder. I’ve never had much luck stitching threads onto contact pads. Conductive thread is better thought of as conductive resister, and as such it’s reasonable at handling low speed signals, that don’t require a great deal signal integrity. The stainless thread that comes with the FLORA is the best I’ve used to date, but it still doesn’t knot very well. This is problematic if you want you connections to stay put as you’re moving around.
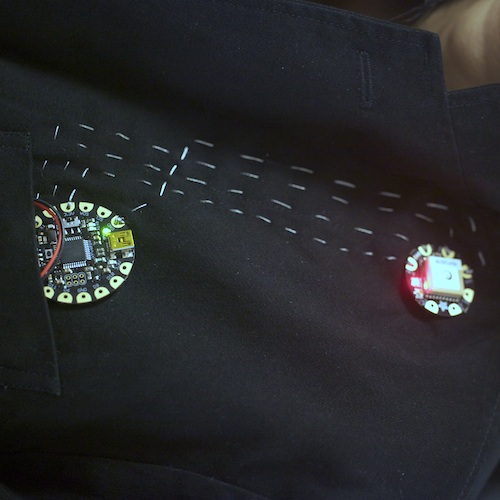
Eventually, after a few short minutes some of the knots I’d put in had come loose and the GPS started dropping packets and power cycling. The terminated connections are also pretty ugly. Little loops of stainless thread. I wanted something a little tidier. I’ve used silver bead crimps in the past for creating reliable connections on surface mount LEDs with great success. Maybe they would work for this project?
The process is pretty simple. Thread a crimp onto the needle and crimp at the far end. Thread through the pad, until the crimp rests flat against the pad. Sew to the other end, and add the other crimp. Fold over onto the pad, solder, and trim. Like so…
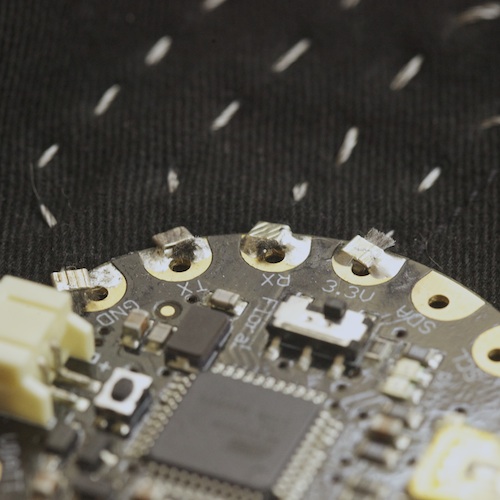
With that sorted, time to make it was time to make the jacket do something. I decided I would make blazer jacket with a boutonnière that would glow brighter the faster I went. One output, and one input. There’s a lot of information you can get out of a GPS: heading, speed altitude, absolute position, time, and so on. The code is really simple:
#include <Adafruit_FloraPixel.h>
Adafruit_FloraPixel strip = Adafruit_FloraPixel(2);
#include <Adafruit_GPS.h>
#include <SoftwareSerial.h>
Adafruit_GPS GPS(&Serial1);
void setup() {
// debug out
Serial.begin(115200);
Serial.println("Go Faster.");
// 9600 NMEA is the default baud rate for Adafruit MTK GPS's- some use 4800
GPS.begin(9600);
GPS.sendCommand(PMTK_SET_NMEA_OUTPUT_RMCGGA);
GPS.sendCommand(PMTK_SET_NMEA_UPDATE_5HZ);
// init the color pixel
strip.begin();
strip.setPixelColor(0, Color(0,16,0));
strip.show();
delay(1000);
}
uint32_t timer = millis();
void loop()
{
// if millis() or timer wraps around, we'll just reset it
if (timer > millis()) timer = millis();
// read the GPS
char c = GPS.read();
if( GPS.newNMEAreceived() )
if( GPS.parse(GPS.lastNMEA()) )
{}
// update the brightness.
if( millis() - timer > 500 )
{
if (GPS.fix)
{
int n = GPS.satellites;
RGBPixel c = Color(8,0,8);
if( n < 5 )
c = Color(0,0,1);
strip.setPixelColor(0, c );
float mph = GPS.speed * 1.15078;
n = mph * 4;
if( mph > 2 )
strip.setPixelColor(0, Color(n,n,n) );
}
else
strip.setPixelColor(0, Color(1,0,0) );
strip.show();
}
}
This works pretty well, and the brightness change between a standstill, walking, and biking is noticeable. The FLORA is small enough that I can hide the GPS, LED, and controller underneath the lapel of the jacket. Until I start moving nothing is noticeable. If I set the threshold to enable the lights above 5mph and add a 15sec fade out timer it turns the jacket into an automatic jogging/bike light, I just need to add shoulder tail lights.
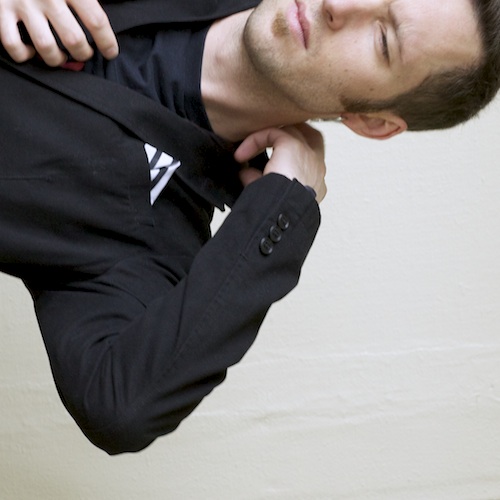
I’ve been thinking about other experiments to do with this jacket. The speedometer is fun, but it wears the batteries down pretty quickly. I’d like to make a jacket that buzzes when I’ve been someplace new. That sort of persistance is difficult to maintain without a lot of support hardware, but I like the idea of have a little reminder to seek out breaks in my daily routine.
LEDs are probably the wrong indicator, a buzzer is better for this sort of thing. Haptic vibration motors are much better at conveying information in clothing. They can be very low power, and generally have a higher environmental contrast ratio than optical systems. That is, when something buzzes against your skin, you notice. As we generally don’t go around staring intently at ourselves, optical systems aren’t as good.
A small haptic output device is one addition to the FLORA platform I’d like to see. Until then, there’s the LilyPad vibe motor that will do the job. It’s a little on the large side, but if you don’t need high resolution or variable intensity it will probably work.